728x90
필수 모듈 설치
ㄴ> pip install pybit
1. session_auth
from pybit import usdt_perpetual
session_auth = usdt_perpetual.HTTP(
endpoint="https://api-testnet.bybit.com",
api_key="your api key",
api_secret="your api secret"
)
2. 주문넣기
#지정가 주문
print(session_auth.place_active_order(
symbol="BTCUSDT",
side="Sell",
order_type="Limit",
qty=0.01,
price=20083,
time_in_force="GoodTillCancel",
reduce_only=False, #True이면 포지션종료 False이면 포지션 오픈
close_on_trigger=False
))
#시장가 주문
print(session_auth.place_active_order(
symbol="BTCUSDT",
side="Buy",
order_type="Market",
qty=0.01,
time_in_force="GoodTillCancel",
reduce_only=False, #True이면 포지션종료 False이면 포지션 오픈
close_on_trigger=False
))
요청 매개변수 ( 매개 변수 필수 유형 설명 )
side | true | string | Side |
symbol | true | string | Symbol |
order_type | true | string | Active order type |
qty | true | number | Order quantity in cryptocurrency |
price | false | number | Order price. Required if you make limit price order |
time_in_force | true | string | Time in force |
reduce_only | true | bool | What is a reduce-only order? True means your position can only reduce in size if this order is triggered. When reduce_only is true, take profit/stop loss cannot be set |
close_on_trigger | true | bool | What is a close on trigger order? For a closing order. It can only reduce your position, not increase it. If the account has insufficient available balance when the closing order is triggered, then other active orders of similar contracts will be cancelled or reduced. It can be used to ensure your stop loss reduces your position regardless of current available margin. |
order_link_id | false | string | Unique user-set order ID. Maximum length of 36 characters |
take_profit | false | number | Take profit price, only take effect upon opening the position |
stop_loss | false | number | Stop loss price, only take effect upon opening the position |
tp_trigger_by | false | string | Take profit trigger price type, default: LastPrice |
sl_trigger_by | false | string | Stop loss trigger price type, default: LastPrice |
position_idx | false | integer | Position idx, used to identify positions in different position modes. Required if you are under One-Way Mode: 0-One-Way Mode 1-Buy side of both side mode 2-Sell side of both side mode |
3. 모든주문취소
print(session_auth.cancel_all_active_orders(
symbol="BTCUSDT" #BTCUSDT 선물 모든 주문 취소
))
4. 조건부 주문
from pybit import usdt_perpetual
session_auth = usdt_perpetual.HTTP(
endpoint="https://api-testnet.bybit.com",
api_key="your api key",
api_secret="your api secret"
)
print(session_auth.place_conditional_order(
symbol="BTCUSDT",
order_type="Limit",
side="Buy",
qty=1,
price=8100,
base_price=16100,
stop_px=8150,
time_in_force="GoodTillCancel",
trigger_by="LastPrice",
order_link_id="cus_order_id_1",
reduce_only=False,
close_on_trigger=False
))
5. 포지션 불러오기
요청
from pybit import usdt_perpetual
session_auth = usdt_perpetual.HTTP(
endpoint="https://api-testnet.bybit.com",
api_key="cCrMK2P55002rmQh1z",
api_secret="eTXOcGvu6Ue9MA916oO5ymqbj2UzBfSLKcti"
)
print(session_auth.my_position(
symbol="BTCUSDT"
))
결과
{
"ret_code": 0,
"ret_msg": "OK",
"ext_code": "",
"ext_info": "",
"result": [
{
"user_id": 533285,
"symbol": "XRPUSDT",
"side": "Buy",
"size": 0,
"position_value": 0,
"entry_price": 0,
"liq_price": 0,
"bust_price": 0,
"leverage": 5,
"auto_add_margin": 0,
"is_isolated": true,
"position_margin": 0,
"occ_closing_fee": 0,
"realised_pnl": 0,
"cum_realised_pnl": -16.35115218,
"free_qty": 0,
"tp_sl_mode": "Full",
"unrealised_pnl": 0,
"deleverage_indicator": 0,
"risk_id": 41,
"stop_loss": 0,
"take_profit": 0,
"trailing_stop": 0,
"position_idx": 1,
"mode": "BothSide"
},
{
"user_id": 533285,
"symbol": "XRPUSDT",
"side": "Sell",
"size": 50,
"position_value": 19.58,
"entry_price": 0.3916,
"liq_price": 0.4529,
"bust_price": 0.4568,
"leverage": 6,
"auto_add_margin": 0,
"is_isolated": true,
"position_margin": 3.2633354,
"occ_closing_fee": 0.013704,
"realised_pnl": 0,
"cum_realised_pnl": 0.90620182,
"free_qty": 50,
"tp_sl_mode": "Full",
"unrealised_pnl": 3.395,
"deleverage_indicator": 2,
"risk_id": 41,
"stop_loss": 0.5,
"take_profit": 0.28,
"trailing_stop": 0,
"tp_trigger_by": 1,
"sl_trigger_by": 1,
"position_idx": 2,
"mode": "BothSide"
}
],
"time_now": "1655868796.293226",
"rate_limit_status": 119,
"rate_limit_reset_ms": 1655868796290,
"rate_limit": 120
}
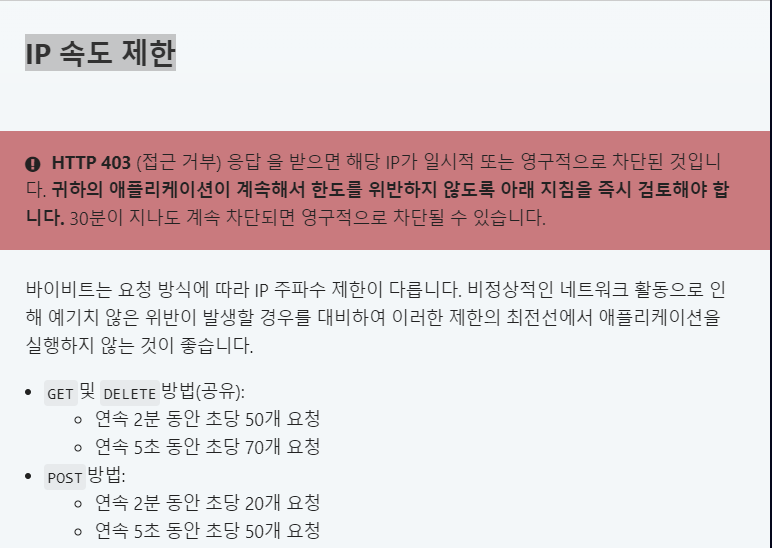
728x90
'블록체인' 카테고리의 다른 글
바이비트 셀퍼럴 | 2023년부터는 거래수수료 돌려받읍시다 / 바이비트 셀퍼럴 방법 (바이비트 레퍼럴 수익 공개) (1) | 2023.01.06 |
---|---|
바이낸스 카피트레이딩 프로그램 봇 소개 feat. 프로그램 무료배포중 (카피트레이딩 수익공개) (0) | 2022.11.23 |
직접 개발한 비트코인 자동매매(feat. Bybit ) 1달간 돌린 결과 공개 / 비트코인 자동매매 프로그램 무료배포 (0) | 2022.09.25 |
업비트 단주매매 프로그램 무료배포 (0) | 2022.07.03 |
신규 P2E게임 백시인피티니 사전예약하시고 100$ 받아가세요 / 액시인피니티 / 무료 에어드랍 (0) | 2021.12.08 |