728x90
잡담
최종 프로젝트 형태는 Pump.fun과 비슷한 Dapp을 배포하는것입니다! 저만의 차별성이 있기도하고 아이디어가 번뜩 떠올라서 구현해보려고합니다. 100개 프로젝트 만들다보면 한개쯤 대박 터지겠죠? 1달에 1개 프로젝트를 만들면 8년안에 pump.fun같은 대박서비스가 개발될수도.... 지금 저는 군대(육군장교)인데 진짜 주간에는
작업하고, 훈련계획짜고, 용사들 챙기고 훈련뛰고나면 개발하기가 힘들지만... 다시 1일1코딩 도전합니다.!
![]() |
![]() |
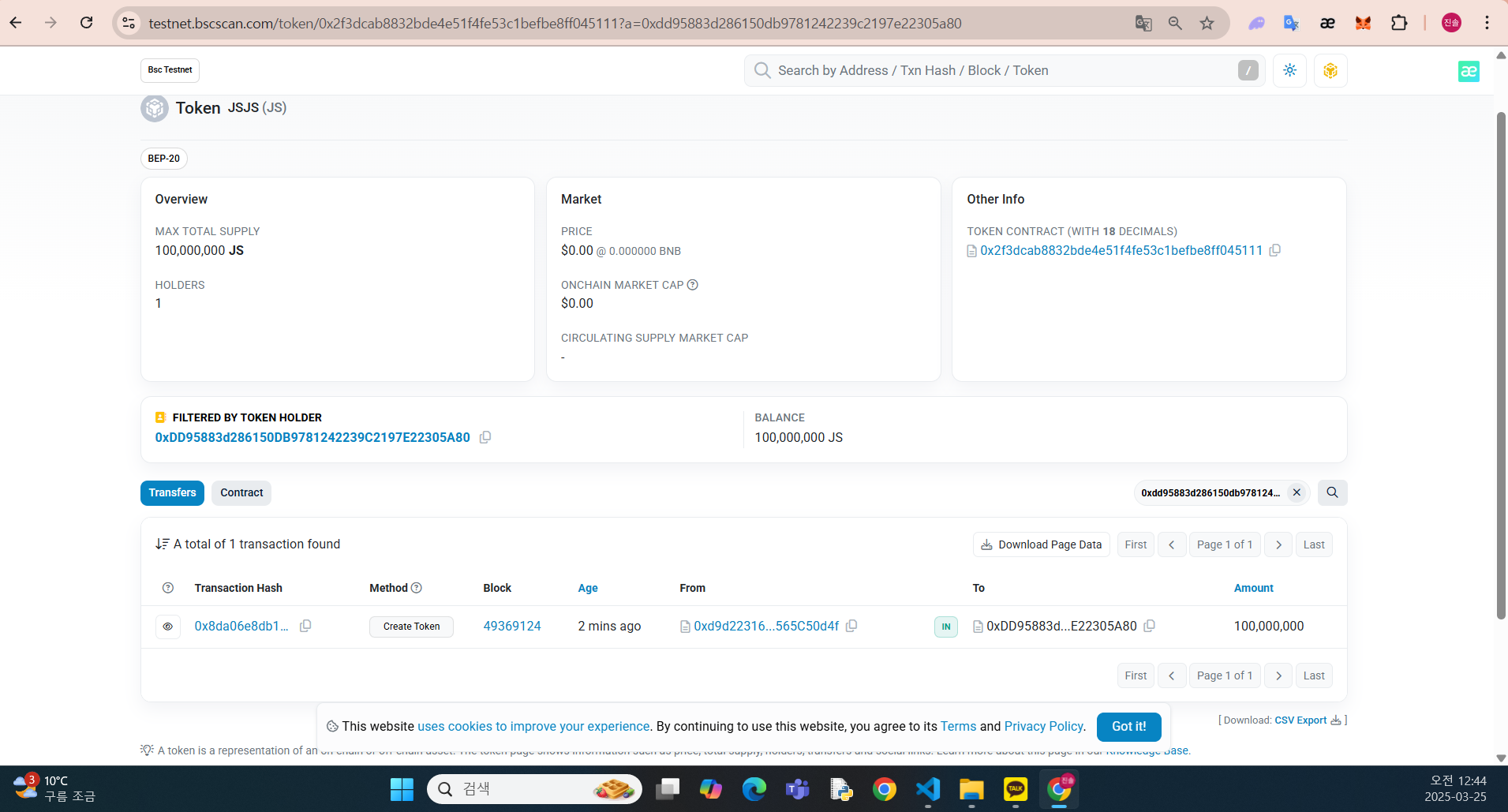
프로젝트 개요
BNB Chain Testnet에서 스마트 컨트랙트를 배포하고, 프론트엔드와 연동해서 토큰 생성 및 프리세일 기능을 구현한 과정을 공유하려고 합니다. Hardhat, React, Ethers.js를 사용했으며, 단계별로 자세히 정리해봤습니다.
이 프로젝트(실습)는 유저가 0.01 BNB(test net bnb)를 지불하면 새로운 ERC-20 토큰을 생성하고, 생성된 토큰을 프리세일 컨트랙트로 전송한 뒤, 프리세일에서 유저가 토큰을 구매할 수 있는 DApp을 만드는 것을 목표로 했습니다. 주요 구성 요소는 다음과 같습니다:
-
TokenFactory 컨트랙트: 새로운 토큰을 생성하고 프리세일 컨트랙트로 전송.
-
Presale 컨트랙트: 프리세일 진행 및 유동성 풀 추가.
-
프론트엔드: React로 구현, 유저가 토큰 생성 및 프리세일에 참여 가능. * ui는 Tailwind Css사용해서 업+Pump.fun과 비슷하게 구현할겁니다!
개발 환경
-
블록체인 네트워크: BNB Chain Testnet
-
스마트 컨트랙트 개발: Hardhat, Solidity
-
프론트엔드: React, Ethers.js
-
지갑: MetaMask
구현 단계
1. 스마트 컨트랙트 작성
1.1 TokenFactory 컨트랙트
TokenFactory 컨트랙트는 유저가 0.01 BNB를 지불하면 새로운 ERC-20 토큰을 생성하고, 발행된 토큰을 Presale 컨트랙트로 전송합니다.
contracts/TokenFactory.sol:solidity
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract TokenFactory is Ownable {
uint256 public constant CREATE_FEE = 0.01 ether;
address public presaleContract;
event TokenCreated(address tokenAddress, string name, string symbol);
constructor(address _presaleContract) Ownable(msg.sender) {
require(_presaleContract != address(0), "Presale contract address cannot be zero");
presaleContract = _presaleContract;
}
function createToken(string memory name, string memory symbol) external payable {
require(msg.value == CREATE_FEE, "Must send 0.01 BNB to create token");
require(bytes(name).length > 0, "Token name cannot be empty");
require(bytes(symbol).length > 0, "Token symbol cannot be empty");
CustomToken newToken = new CustomToken(name, symbol, 100_000_000 * 10**18);
require(newToken.transfer(presaleContract, 100_000_000 * 10**18), "Token transfer failed");
emit TokenCreated(address(newToken), name, symbol);
}
function withdraw() external onlyOwner {
payable(owner()).transfer(address(this).balance);
}
}
contract CustomToken is ERC20 {
constructor(string memory name, string memory symbol, uint256 initialSupply)
ERC20(name, symbol)
{
_mint(msg.sender, initialSupply);
}
}
1.2 Presale 컨트랙트
Presale 컨트랙트는 유저가 BNB를 보내서 토큰을 구매할 수 있도록 하고, 목표 BNB(5 BNB)에 도달하면 PancakeSwap에 유동성을 추가합니다.
contracts/Presale.sol (일부 생략):solidity
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract Presale is Ownable {
IERC20 public token;
uint256 public constant INITIAL_RATE = 10_000_000;
uint256 public constant TARGET_BNB = 5 ether;
uint256 public totalBNB;
mapping(address => uint256) public contributions;
mapping(address => uint256) public tokenBalances;
constructor(IERC20 _token) Ownable(msg.sender) {
token = _token;
}
function buyTokens() external payable {
uint256 tokenAmount = msg.value * INITIAL_RATE;
require(token.balanceOf(address(this)) >= tokenAmount, "Not enough tokens");
contributions[msg.sender] += msg.value;
tokenBalances[msg.sender] += tokenAmount;
totalBNB += msg.value;
}
}
2. 스마트 컨트랙트 배포
Hardhat을 사용해 BNB Chain Testnet에 컨트랙트를 배포했습니다.
scripts/deploy.js:javascript
const hre = require("hardhat");
async function main() {
const [deployer] = await hre.ethers.getSigners();
console.log("Deploying contracts with the account:", deployer.address);
const CustomToken = await hre.ethers.getContractFactory("CustomToken");
const testToken = await CustomToken.deploy("Test Token", "TST", ethers.parseEther("100000000"));
await testToken.waitForDeployment();
console.log("Test Token deployed to:", await testToken.getAddress());
const Presale = await hre.ethers.getContractFactory("Presale");
const presale = await Presale.deploy(await testToken.getAddress());
await presale.waitForDeployment();
console.log("Presale deployed to:", await presale.getAddress());
const TokenFactory = await hre.ethers.getContractFactory("TokenFactory");
const tokenFactory = await TokenFactory.deploy(await presale.getAddress());
await tokenFactory.waitForDeployment();
console.log("TokenFactory deployed to:", await tokenFactory.getAddress());
}
main().catch((error) => {
console.error(error);
process.exitCode = 1;
});
배포 명령어:bash
npx hardhat run scripts/deploy.js --network bscTestnet
배포 결과:(테스트넷)
-
Test Token: 0x8721C944f7Eb09F81FA12527ee6f7eea4A121696
-
Presale: 0xDD95883d286150DB9781242239C2197E22305A80
-
TokenFactory: 0xd9d2231675C43a4751AE5d262Ee54bc565C50d4f
3. 프론트엔드 구현
React와 Ethers.js를 사용해 프론트엔드를 구현했습니다. 유저가 토큰을 생성할 수 있는 모달을 만들었어요.
3.1 토큰 생성 모달
frontend/src/components/CreateTokenModal.js (간략화된 버전):
javascript
import React, { useState } from "react";
import { ethers } from "ethers";
import TokenFactoryABI from "../abis/TokenFactory.json";
const TOKEN_FACTORY_ADDRESS = "0xd9d2231675C43a4751AE5d262Ee54bc565C50d4f";
const CreateTokenModal = ({ onClose, onCreate, signer }) => {
const [name, setName] = useState("");
const [symbol, setSymbol] = useState("");
const [error, setError] = useState("");
const handleSubmit = async () => {
if (!signer) {
setError("Please connect your wallet first.");
return;
}
try {
const tokenFactory = new ethers.Contract(
TOKEN_FACTORY_ADDRESS,
TokenFactoryABI,
signer
);
const tx = await tokenFactory.createToken(name, symbol, {
value: ethers.parseEther("0.01"),
gasLimit: 1000000,
});
await tx.wait();
onCreate({ name, symbol });
onClose();
} catch (err) {
setError("Transaction failed: " + err.message);
}
};
return (
<div className="fixed inset-0 bg-black bg-opacity-70 flex items-center justify-center">
<div className="bg-gray-800 p-6 rounded-lg w-full max-w-md shadow-xl">
<h2 className="text-xl font-semibold mb-4">Create New Token</h2>
{error && <div className="mb-4 p-2 bg-red-600 text-white rounded">{error}</div>}
<div className="mb-4">
<label className="block mb-1 text-gray-300">Token Name</label>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
className="w-full p-2 bg-gray-700 rounded border border-gray-600"
placeholder="My Token"
/>
</div>
<div className="mb-4">
<label className="block mb-1 text-gray-300">Token Symbol</label>
<input
type="text"
value={symbol}
onChange={(e) => setSymbol(e.target.value)}
className="w-full p-2 bg-gray-700 rounded border border-gray-600"
placeholder="MTK"
/>
</div>
<div className="flex justify-end space-x-3">
<button onClick={onClose} className="bg-gray-600 px-4 py-2 rounded-lg">
Cancel
</button>
<button onClick={handleSubmit} className="bg-blue-600 px-4 py-2 rounded-lg">
Create (0.01 BNB)
</button>
</div>
</div>
</div>
);
};
export default CreateTokenModal;
3.2 테스트
프론트엔드를 실행하고, MetaMask를 연결한 뒤 토큰 생성을 테스트했습니다.
명령어:
bash
cd frontend
npm start
-
"Create Token" 버튼 클릭 → 이름: "MyToken", 심볼: "MTK" 입력.
-
0.01 BNB 지불 → 트랜잭션 성공 → 토큰 생성 완료.
4. 토큰 소유자 확인
TokenFactory에서 생성된 토큰은 Presale 컨트랙트로 전송됩니다. 따라서 현재 1억 토큰은 Presale 컨트랙트(0xDD95883d286150DB9781242239C2197E22305A80)가 소유하고 있습니다.
마무리
오늘은 BNB Chain Testnet에서 토큰 생성 및 프리세일 DApp의 기본 기능을 구현해봤습니다. 몇 가지 에러(예: 트랜잭션 리버트, 가스 한도 문제)를 해결하면서 많은 것을 배웠어요. 다음 단계로는 프리세일 페이지에서 유저가 토큰을 구매할 수 있도록 기능을 추가할 예정입니다.
728x90
'Solidity' 카테고리의 다른 글
2. BNB 토큰발행 및 프리세일 구현(구매 및 환불) (Solidity Project Day2~4) + React (0) | 2025.03.31 |
---|---|
Truffle require('chai') Error [ERR_REQUIRE_ESM 해결방법 (1) | 2024.02.24 |
Solidity Tutorial lec.2 / 함수제어자, 예외처리, 전역변수msg (0) | 2024.02.18 |
Solidity Tutorial lec.1 / Solidity란, 스마트계약, Solidity 문법 (0) | 2024.02.16 |